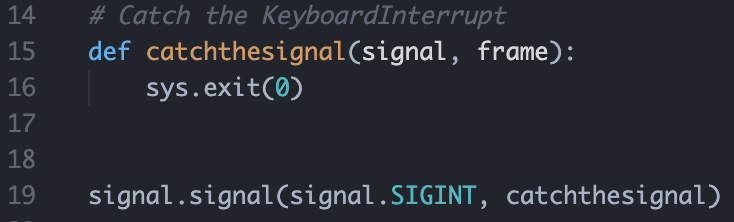
CONTROL + C is the magic key combination to stop any execution at the command line. The execution stops immediately and in worst case you see something like ^C as the output of your running command.
If you stop the execution of any Python-Program, the output will be a bit unordinary and in the most cases, annoying. Because your Terminal gets flooded and you will get an Exception:
^CTraceback (most recent call last):
File "garbage_collector.py", line 5, in <module>
time.sleep(200)
KeyboardInterrupt
In this example you see both of the described outputs the interruption ^C and the python exception. Personally I don’t like this kind of exception and always keep the eye on it to prevent the output of KeyboardInterrupt-Exception.
The following ways are really good for production and non production usage:
#!/usr/bin/env python3
# Header of the python script
import sys
import [..]
# Catch the KeyboardInterrupt
def catchthesignal(signal, frame):
sys.exit(0)
signal.signal(signal.SIGINT, catchthesignal)
# The main logic of the script
...
The less elegant way is to use a try/except around your main logic, but this works not always as expected so the above example works like a charm :)
try:
while True:
main_logic()
except KeyboardInterrupt:
print("KeyboardInterrupt has been catched!")
Happy pythoning!
you can try this one solution:
try:
while True:
time.sleep(120)
except KeyboardInterrupt:
print("\r ")
print("KeyboardInterrupt has been catched!")
Is there any way to prevent this “^C” from being printed on the terminal?