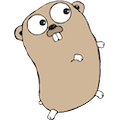
Golang short Go is an open source programming language, which was invented in 2007. Go makes it easy to build simple, reliable, and efficient software.
Go as a language is more similar to C.
Installation on MAC OS X
brew update
brew install golang
Installation on CentOS
Download the latest golang version from official golang website. (make sure you have whet or curl installed)
wget https://golang.org/dl/go1.15.6.linux-amd64.tar.gz
# extract archive to /usr/local
tar -C /usr/local -xzf go1.15.6.linux-amd64.tar.gz
# Write path to ~/.bash_profile
echo "export PATH=\$PATH:/usr/local/go/bin" >> ~/.bash_profile
After successful installation run:
go version
to verify the version of the installed golang.
golang workspace
Now it’s time to organise the working space for golang and the environment for the first hello world example.
# golang env, from ~/.bash_profile
export GOPATH=$HOME/devel/go-workspace
export GOBIN=$HOME/devel/go-workspace/bin
export GOROOT=/usr/local/go
export PATH=$PATH:$GOPATH/bin
export PATH=$PATH:$GOROOT/bin
Restart the session and based to reload the environment and create required directories:
mkdir -p $GOPATH $GOPATH/src $GOPATH/pkg $GOPATH/bin
Create the first source code for the hello world program:
$GOPATH/src/hello.go
Source code of hello.go:
package main
import "fmt"
func main() {
fmt.Println("Hello, world.")
}
Run hello.go program:
go run $GOPATH/src/hello.go
Compile hello.go, the binary will be placed automatically to $GOPATH/bin/
go install $GOPATH/src/hello.go
Previously we set $PATH to $GOPATH/bin so we are able to execute go binaries by running them by name:
hello
Source code formatting
Golang has a build in source code formatter:
gofmt -w $GOPATH/src/hello.go
which makes your code human readable and correct the formatting. Really a great solution.
Maybe you have checked the size of the hello binary after compilation, don’t be confused about the size of around 1.9M.
The linkers in the gc tool chain do static linking. So all Go binaries therefore include the Go run-time.
ARM ready
Compile go source for ARM processor units:
env GOOS=linux GOARCH=arm GOARM=5 go install $GOPATH/src/hello.go
Now it’s possible to run hello on any ARM chip system.
Example for arguments
package main
import (
"fmt"
"os"
)
func main() {
if len(os.Args) > 1 {
my_command := os.Args[1:]
fmt.Println(my_command)
} else {
fmt.Println("Error: no arguments were specified")
os.Exit(0)
}
}
Example for system calls
package main
import (
"os/exec"
)
func main() {
command := "sleep"
my_exec := exec.Command(command, "5")
my_exec.Output()
}
Leave a Reply